How to Use Hooks in Laravel
Laravel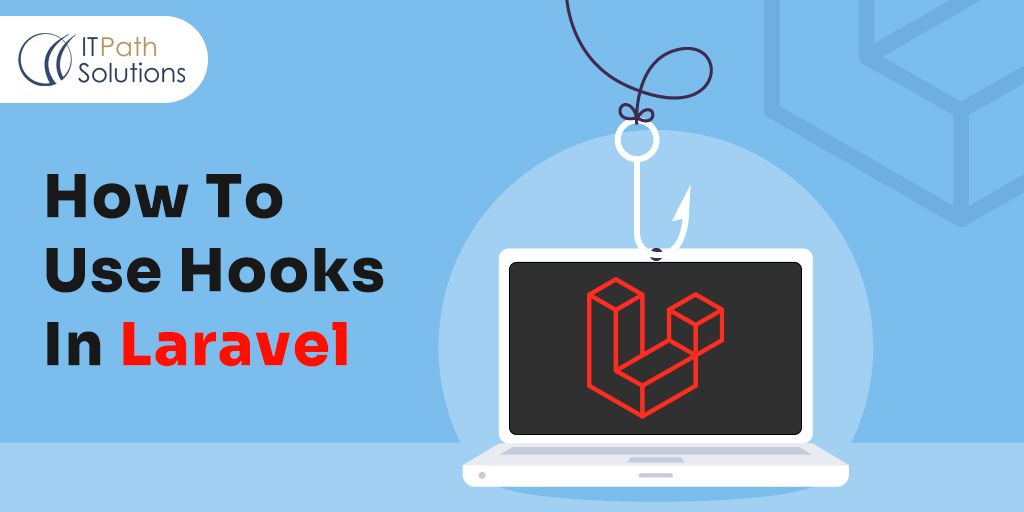
In Laravel development, hooks play a vital role in extending the framework’s functionality and customizing application behavior. Hooks, also known as events or event hooks, are points in the application’s execution flow where developers can inject custom code to modify or enhance the default behavior of Laravel.
The significance of hooks in Laravel development is manifold. They provide a flexible and modular approach to modify application behavior without directly modifying the core framework code. This allows developers to customize Laravel applications to meet specific business requirements while adhering to best practices and maintaining code integrity.
Hooks in Laravel enable developers to listen for specific events or actions triggered during the application’s execution, such as before or after a database transaction, before rendering a view, or after a user authentication. By attaching custom code to these hooks, developers can introduce additional functionality, perform specific actions, modify data, or interact with external systems.
Understanding Hooks in Laravel
Hooks in Laravel are event-driven mechanisms that allow developers to intercept and modify the application’s behavior at specific points in its execution flow. They provide a way to extend the functionality of Laravel without directly modifying the core framework code. Hooks, also referred to as events or event hooks, allow developers to attach custom code to predefined events and execute that code when the corresponding event is triggered.
In Laravel, hooks are implemented using the built-in event system. The event system consists of event classes, event listeners, and event dispatchers. Developers define events as classes, which contain relevant data and are responsible for triggering the event. Event listeners, on the other hand, are classes that contain the custom code to be executed when a specific event is fired. The event dispatcher is responsible for connecting events and listeners, dispatching events, and executing the associated listeners.
While both middleware and hooks serve to intercept and modify the application’s behavior, there are some key differences between the two in the context of Laravel.
Middleware in Laravel acts as a series of filters that are applied to incoming requests or outgoing responses. Middleware operates on the HTTP layer and is executed sequentially for each request. It can perform tasks such as authentication, authorization, request validation, and more. Middleware typically acts on the entire request-response cycle and can terminate the request or modify the response.
Hooks, on the other hand, are event-based mechanisms that allow developers to modify the application’s behavior at specific points in its execution flow. Hooks are typically associated with more granular events within the application, such as before or after a database transaction, before rendering a view, or after a user authentication. Hooks are not limited to the HTTP layer and can be triggered by various events throughout the application’s lifecycle.
Benefits of Using Hooks in Laravel Applications
- Extensibility: Hooks provide a flexible way to extend the functionality of Laravel without modifying the core framework code. Developers can attach custom code to hooks to introduce additional features or modify existing behavior according to specific requirements.
- Code Reusability: By separating custom code into hooks, developers can reuse the same code across different parts of the application. This promotes code reusability, reduces redundancy, and simplifies maintenance and updates.
- Modular Approach: Hooks allow developers to decouple code and modularize the application’s functionality. This improves code organization, readability, and makes it easier to manage and understand the flow of the application.
- Interoperability: Hooks facilitate seamless integration with third-party libraries, services, or APIs. Developers can use hooks to trigger external actions, interact with external systems, or communicate with other applications when specific events occur.
- Customization: Hooks provide a way to customize the behavior of the application based on specific business requirements. Developers can attach custom code to hooks to perform specific actions, modify data, or implement complex workflows without disrupting the core functionality.
- Scalability: By utilizing hooks, developers can add new features or modify existing behavior without impacting the overall performance and scalability of the application. Hooks enable developers to introduce changes at specific points in the application’s execution flow, ensuring efficient and optimized performance.
Types of Hooks in Laravel
Pre-hooks: Exploring hooks executed before a specific action
Pre-hooks in Laravel are hooks that are executed before a specific action or event takes place within the application. These hooks allow developers to intercept and modify the data, perform validations, or execute custom code just before the actual action occurs. Pre-hooks are commonly used to enforce business rules, validate user input, or modify data before it is saved to the database.
For example, a pre-hook can be used to validate user input in a registration form before creating a new user record. The pre-hook can check if the required fields are filled, validate the email format, or perform any custom validations. By executing the pre-hook before the actual registration process, developers can ensure that only valid data is processed, improving the overall data integrity and user experience.
Post-hooks: Exploring hooks executed after a specific action
Post-hooks in Laravel are hooks that are executed after a specific action or event has occurred within the application. These hooks provide developers with the opportunity to perform additional tasks, modify data, or trigger further actions based on the outcome of the original action. Post-hooks are commonly used for logging, sending notifications, or performing actions that depend on the result of a previous operation.
For example, after a user successfully submits a form, a post-hook can be used to send a confirmation email or trigger a notification to the user or an administrator. The post-hook can access the submitted data, generate the email content, and send it using the appropriate email service. This allows developers to enhance user engagement and provide timely feedback after the completion of a specific action.
Conditional hooks: Exploring hooks executed based on certain conditions
Conditional hooks in Laravel are hooks that are executed based on specific conditions or criteria. These hooks allow developers to control when and where the custom code is executed based on various factors such as user roles, environment settings, or specific data conditions. Conditional hooks provide a powerful mechanism to implement complex logic and customize the application’s behavior based on dynamic conditions.
For example, a conditional hook can be used to perform additional processing on a user’s data only if they belong to a specific user role or have met certain criteria. The conditional hook can check the user’s role, query additional data, or evaluate conditions before executing the custom code. This enables developers to tailor the application’s behavior to different user segments or specific scenarios.
Global hooks: Exploring hooks that are applicable across the entire application
Global hooks in Laravel are hooks that are applicable across the entire application, regardless of the specific action or event. These hooks are executed at key points in the application’s lifecycle and provide developers with a centralized mechanism to implement common functionality or enforce global behaviors.
For example, a global hook can be used to log every database query executed by the application. By attaching the hook to the database query event, developers can capture and store information about each query, such as the SQL statement, execution time, or the user who initiated the query. This allows for comprehensive monitoring, auditing, and performance analysis of the application’s database interactions.
In summary, Laravel offers various types of hooks that allow app developers to extend, customize, and control the application’s behavior. Pre-hooks and post-hooks enable actions to be performed before and after specific events, while conditional hooks provide flexibility based on specific conditions. Global hooks offer a centralized mechanism to implement common functionality across the entire application. By utilizing these different types of hooks effectively, developers can create highly customizable, flexible, and feature-rich Laravel applications.
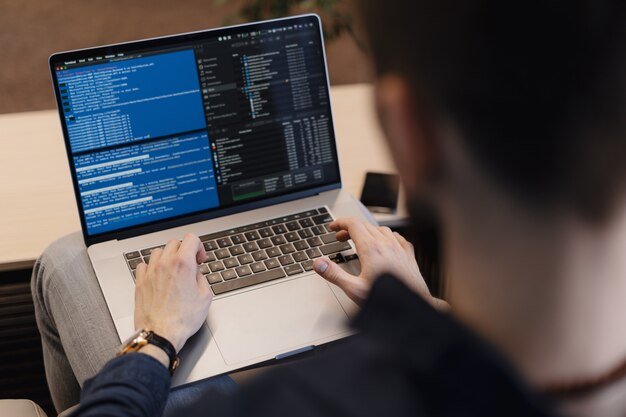
Implementing Hooks in Laravel
Setting up Laravel project and environment
To implement hooks in Laravel, start by setting up a Laravel project and configuring the development environment. Install Laravel using Composer, set up the database connection, and configure the necessary environment variables. Ensure that you have a functional Laravel installation to proceed with implementing hooks effectively.
Creating custom hooks using Laravel’s built-in event system
Laravel provides a robust event system that serves as the foundation for implementing hooks. Begin by creating custom event classes that represent the specific events you want to hook into. These event classes should extend the Illuminate\Foundation\Events\Event class and define any necessary properties or data related to the event.
For example, you can create a UserRegistered event class that contains information about a user who has successfully registered. Within this class, define the necessary properties such as user details, registration timestamp, or any additional data you want to pass along with the event.
Registering and defining hooks in the application
To utilize the custom hooks, register event listeners that will be executed when the associated events are fired. In Laravel, event listeners are classes that contain the custom code you want to execute when a specific event occurs. These listeners should implement the Illuminate\Contracts\Events\Listener interface and define the handle() method to perform the desired actions.
For our UserRegistered event, create a corresponding SendWelcomeEmail listener class. In the handle() method, write the logic to send a welcome email to the newly registered user. Within this listener class, you can access the event data and perform any necessary operations.
After creating the event listeners, register them in Laravel’s event service provider. This can be done in the EventServiceProvider class, where you can associate the events with their corresponding listeners using the listen() method.
Demonstrating how to execute hooks at different stages of the request lifecycle
Laravel provides various points in the request lifecycle where hooks can be executed. These stages include the bootstrap process, middleware execution, and response generation.
For example, you can create a hook that runs during the bootstrap process to perform tasks such as environment configuration, loading service providers, or initializing custom components. You can achieve this by registering the hook in the bootstrap/app.php file.
Hooks can also be executed during middleware execution, allowing you to modify the request or response data before reaching the route handler. By creating custom middleware classes and attaching them to specific routes or route groups, you can execute hooks at desired stages of the middleware pipeline.
Finally, you can implement hooks during the response generation phase. This allows you to modify the response data or perform additional tasks before sending the response to the client. Laravel provides the Terminating event, which fires just before the response is sent. Register a listener for this event to execute any necessary post-processing or logging tasks.
In summary, implementing hooks in Laravel involves creating custom event classes, defining event listeners, registering the listeners in the event service provider, and executing the hooks at different stages of the request lifecycle. By utilizing Laravel’s event system, you can extend and customize the framework’s behavior, perform additional tasks, and integrate custom functionality seamlessly into your Laravel application.
Real-World Examples of Using Hooks in Laravel
Authentication hooks: Implementing hooks for user authentication
Hooks can be used to enhance the authentication process in Laravel. For example, you can create a pre-hook that runs before the authentication process to perform additional checks or validations. This can include verifying the user’s email status, checking for account suspension, or implementing custom authentication rules. By utilizing authentication hooks, you can extend Laravel’s default authentication behavior and add custom logic to ensure a secure and tailored authentication experience for your users.
Authorization hooks: Implementing hooks for user permissions and access control
Hooks can also be employed for implementing user permissions and access control in Laravel applications. You can create conditional hooks that run before accessing specific routes or performing certain actions. These hooks can check the user’s role or permissions, validate access rights, or implement custom authorization rules. By utilizing authorization hooks, you can customize the authorization logic to align with your application’s specific requirements and ensure that users only have access to the resources they are authorized to use.
Logging hooks: Implementing hooks to log specific actions or events
Hooks are valuable for implementing logging functionality in Laravel applications. You can create post-hooks that run after critical actions or events, such as user registrations, data updates, or payment transactions. These hooks can be used to log relevant information, such as the user’s IP address, the performed action, or the executed SQL queries. By implementing logging hooks, you can easily track and monitor important activities in your application, improve debugging capabilities, and maintain an audit trail of significant events.
Integration hooks: Implementing hooks for integrating with external services or APIs
Hooks can facilitate the integration of Laravel applications with external services or APIs. You can create post-hooks that execute after specific events, such as successful form submissions or order placements. These hooks can be utilized to trigger actions in external systems, such as sending data to a CRM, initiating email notifications, or updating records in a third-party application. By leveraging integration hooks, you can seamlessly connect your Laravel application with other services, automate processes, and enhance overall system integration and data synchronization.
In conclusion, hooks in Laravel offer a wide range of possibilities for extending, customizing, and enhancing your application’s functionality. Real-world examples include using hooks for authentication and authorization, implementing logging capabilities, and integrating with external services. By leveraging hooks effectively, you can tailor your Laravel application to meet specific requirements, streamline processes, and provide an optimal user experience.
Advanced Techniques and Tips
Chaining hooks for complex scenarios
Laravel’s hooks can be chained together to handle more complex scenarios. You can create multiple hooks that are executed in a specific order, allowing you to perform a series of actions or validations. For example, you can create a pre-hook that validates user input, followed by a conditional hook that checks certain conditions, and finally, a post-hook that performs additional processing or triggers specific events. By chaining hooks, you can build a flexible and robust workflow that accommodates intricate business logic and ensures smooth execution of complex operations.
Handling exceptions and error handling within hooks
When working with hooks in Laravel, it’s crucial to handle exceptions and errors gracefully. You can utilize Laravel’s exception handling mechanisms within hooks to catch and handle errors effectively. This includes using try-catch blocks, logging exceptions, and providing appropriate error messages to users. By implementing proper exception handling, you can ensure that your application remains stable and resilient even when encountering unexpected issues within hooks.
Extending Laravel’s hooks functionality using custom packages
Laravel’s hooks functionality can be extended further by leveraging custom packages. These packages can provide additional hooks, utilities, or integrations that cater to specific needs. You can explore Laravel’s package ecosystem and choose packages that align with your project requirements. By incorporating custom packages, you can enhance the capabilities of Laravel’s hooks system, save development time, and leverage pre-built solutions to tackle common challenges in your application.
Performance considerations when using hooks in Laravel
While hooks can greatly enhance the flexibility and functionality of your Laravel application, it’s essential to consider performance implications. Hooks that execute complex operations or make numerous database queries can impact application performance. Therefore, it’s important to optimize the execution of hooks by minimizing unnecessary operations, utilizing caching mechanisms, and implementing efficient database queries. Additionally, monitor the performance of your application using Laravel’s profiling tools or third-party monitoring solutions to identify and address any performance bottlenecks introduced by hooks.
Conclusion
Integrating hooks in Laravel provides developers with a powerful mechanism to extend and customise the framework’s behaviour. By leveraging hooks, developers can enhance data management, streamline workflows, and integrate with external services seamlessly. The flexibility offered by hooks allows for the implementation of complex scenarios, advanced error handling, and the ability to optimise performance. Whether it’s authentication, authorization, logging, or integration, hooks in Laravel empower developers to create feature-rich applications tailored to specific requirements. Embracing hooks in Laravel development opens up a world of possibilities for building robust and efficient web applications.