A Quick Guide To SwiftUI Modifiers
iOS App Development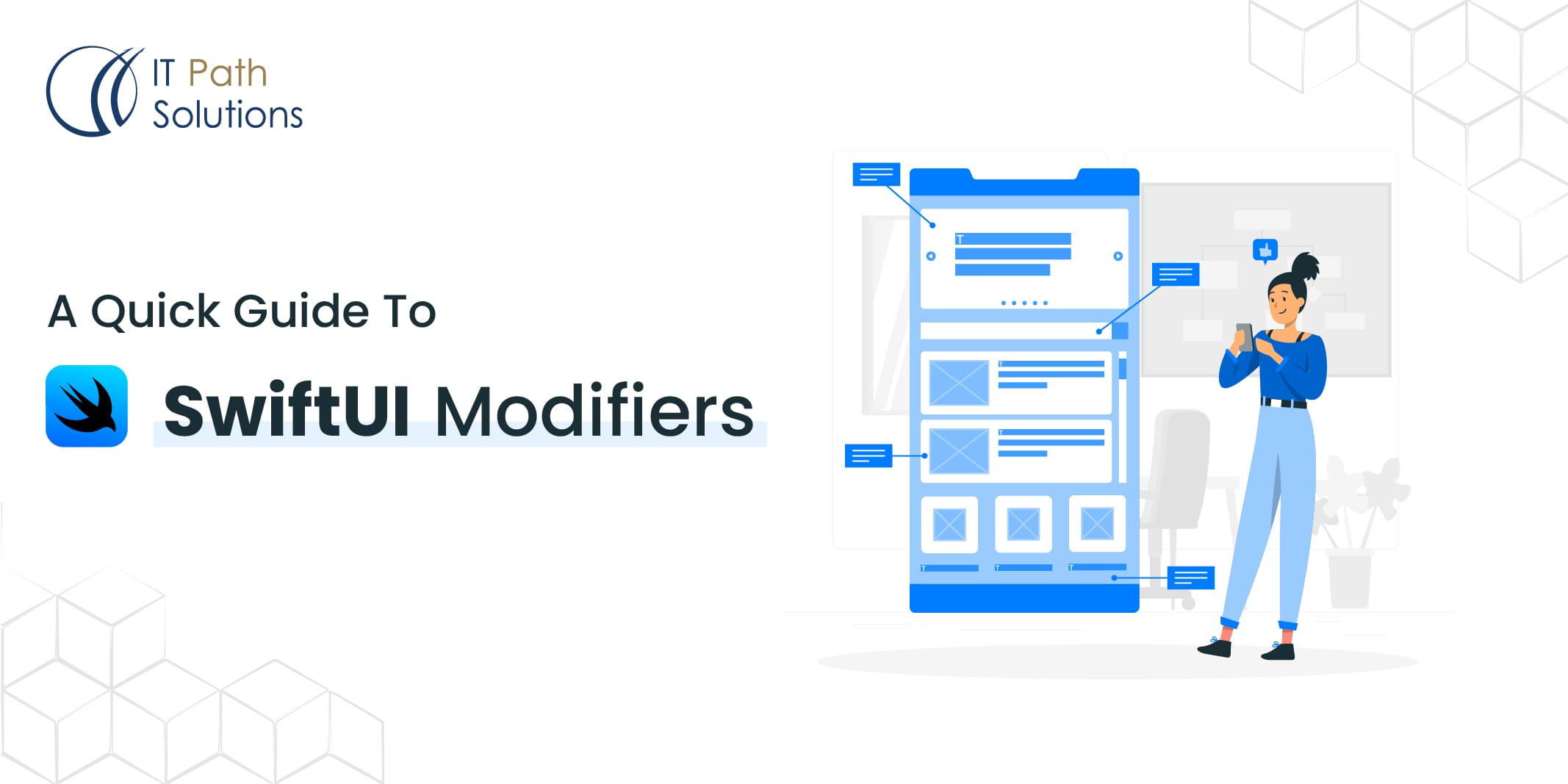
Introduction
Creating compelling user interfaces is a paramount goal in app development. It’s crucial to engage users and provide them with intuitive experiences. In this pursuit, Apple’s SwiftUI has emerged as a game-changer, offering a declarative UI framework that simplifies the process while providing adaptability and robust capabilities.
One of the key tools in SwiftUI’s arsenal that has garnered significant attention is SwiftUI Modifiers. These modifiers play a pivotal role in customizing and enhancing both the visual and functional aspects of UI components.
Understanding SwiftUI Modifiers
SwiftUI Modifiers are a foundational concept that empowers iOS App developers to alter the attributes of UI elements, encompassing elements like text views, buttons, and images. The hallmark of modifiers lies in their chainable nature; they can be combined sequentially to conjure desired visual and interactive effects. Each modifier is a lightweight function, accepting an input, transforming it, and returning a refined output. This philosophy seamlessly aligns with SwiftUI’s declarative syntax, enabling developers to articulate the UI’s intended appearance rather than the intricate steps to manifest it.
Commonly Used Modifiers
- font(): This modifier wields the power to tweak font style and size of textual elements. Employing this modifier on a Text view can bestow it with a unique typographical flair.
- foregroundColor(): The ability to alter the hue of text and other UI elements is effortlessly achieved using this modifier. It’s a potent instrument for enhancing the visual hierarchy and conveying significance through color.
- padding(): For a tidy and organized UI, the padding modifier steps in, permitting meticulous control over element spacing, thus achieving equilibrium in the layout.
- background(): Casting a transformative spell on a view’s background is the objective of this modifier. It’s akin to an artistic canvas where colors, gradients, images, or even fellow views can paint the backdrop.
- cornerRadius(): Modernity and sophistication converge as UI elements acquire sleek, rounded edges through this modifier. The transformation is particularly impactful for buttons and image views.
- onTapGesture(): Modifiers aren’t confined to appearances; they also hold sway over behavior. The onTapGesture modifier confers interactivity to views, enabling the definition of actions for user taps.
- rotationEffect(): Infuse dynamism into your UI using this modifier’s rotation effect. It’s a valuable tool for crafting captivating transitions and visual spectacles.
What Is A View Modifier?
A ViewModifier is a construct in SwiftUI that takes an existing view, applies specific modifications to it, and then returns the modified view as a result. It’s a way to customize the appearance or behavior of a view. To create our own custom view modifier, we define a new type that conforms to the ViewModifier protocol. This custom type encapsulates the modifications we want to apply to views, allowing us to reuse these modifications across different parts of our SwiftUI code.
Here’s my first go at creating a Caption view modifier
Fig 2. Caption view modifier
The primary requirement when creating a custom view modifier is to implement the body method. This method takes in some content (a view) and is responsible for returning a modified view. In this case, you can take inspiration from the modifiers used in the country view and apply them to the content within your custom modifier. We’ll explore how to customize the font shortly.
We can apply our Caption modifier to a view using modifier()
A more elegant approach is to extend the View type by adding our custom modifier as an extension. This way, we can directly apply our Caption modifier to any view, resulting in cleaner and more intuitive code.
Fig 3. Extension view
Now, we have the convenience of applying our custom modifier directly to a view, just like we do with the standard SwiftUI modifiers. This makes our code more consistent and straightforward.
Fig 4. Caption applied
Custom Modifiers
A custom SwiftUI view modifier is a way to encapsulate a set of view modifiers that you want to reuse across multiple views in your SwiftUI app. This helps eliminate duplication and enhances the readability of your cod
Certainly, let’s start with our SwiftUI view containing two text views with some styling applied.
Fig 5. Text Modifiers integrated using SwiftUI
To support both light and dark modes in our SwiftUI view, we can use SwiftUI’s built-in support for color schemes. Here’s how our view would look in both light and dark modes.
Fig 6. Output After applying Text Modifier
In my SwiftUI view, I’ve identified repetitive elements. The two text views share the same four view modifiers: font (with different values), background, padding, and corner radius. To enhance code organization and readability, we can consolidate these shared modifiers into a custom modifier of our own. This custom modifier can then be readily applied to any view, simplifying our code and eliminating redundancy.
Functional Approach
Indeed, Modifiers and Stacks share a common characteristic: they both encapsulate or wrap content. Stacks achieve this by utilizing a closure to enclose their content. One might naturally wonder why not employ a similar approach for modifiers, encapsulating their modifications within a closure.
Fig 7. Modifier using closure
I personally find this approach more intuitive in certain scenarios because it simplifies the understanding of how a modifier is applied. However, there’s a potential issue with this method: when we start stacking multiple modifiers, it can lead to what’s often called a “Pyramid of Doom.” In other words, it becomes progressively harder to manage and read the code as we add more and more modifiers, diminishing its clarity and maintainability.
Fig 8. Applied FrameLayout Modifier
The functional approach chosen is much more scalable and cleaner and once you get used it becomes clear that it’s the right choice.
Fig 9. Applied Modifier to Rectangle
Fig 10. Reasons to use SwiftUI Modifiers
Declarative Syntax
SwiftUI modifiers enable a highly declarative syntax for designing UIs. we specify what we want our UI to look like, and the modifiers handle the implementation details.
Readability:
By chaining modifiers, we can create a clear and readable flow of code that describes the appearance and behavior of our UI components.
Fig 11. Readability
By chaining modifiers in a clear sequence, it’s easy to understand the appearance and behavior of each UI component.
Reusability:
Modifiers encourage the creation of reusable components. We can define a set of modifiers that encapsulate a certain style or behavior and apply them to multiple views.
Fig 12. Reusability
We can now apply the RoundedButtonStyle modifier to multiple buttons for consistent styling.
Separation of Concerns:
Modifiers allow us to separate concerns within our UI code. We can focus on the layout and structure of our views and then add modifiers to control appearance and behavior.
Fig 13. Separation of Concerns
Separating the header and body views allows us to focus on their individual layouts and apply modifiers independently.
Dynamic Updates:
Modifiers automatically update our UI when the underlying data changes. This makes it easy to create reactive and responsive interfaces.
Composability:
SwiftUI’s composability shines through modifiers. We can combine multiple modifiers to create complex effects without needing to write extensive code. We can combine modifiers to create complex effects. For instance, combining .rotationEffect and .scaleEffect can create a rotating and scaling animation.
Animation:
Modifiers make it simple to add animations to our UI. We can animate changes to view properties like position, opacity, and scale with just a few lines of code.
Fig 24. Animation
RoundedRectangle’s corner radius, opacity, and scale change with a smooth animation. The .animation modifier is used to specify the animation type and duration.
Accessibility:
SwiftUI provides accessibility modifiers that enhance the accessibility of your app for users with disabilities. This includes adjusting labels, hints, and traits for UI elements. SwiftUI provides accessibility modifiers to enhance accessibility. For instance, you can set accessibility labels and hints.
Consistency:
SwiftUI modifiers help you maintain a consistent visual style throughout your app by applying the same set of modifiers to different views.
Fig 26. Consistency
Apply the same set of modifiers to maintain a consistent style throughout our app.
Performance:
SwiftUI’s layout system optimizes rendering by efficiently applying changes made through modifiers. This can lead to improved performance compared to traditional imperative UI frameworks.
SwiftUI’s layout system efficiently applies changes through modifiers, optimizing rendering for improved performance compared to imperative UI frameworks. This optimization happens automatically behind the scenes.
Conclusion
SwiftUI Modifiers serve as the cornerstone of SwiftUI development, affording Swift developers the capability to orchestrate captivating and interactive user interfaces with remarkable ease. A nuanced comprehension of modifier fundamentals coupled with a voyage into their diverse applications empowers you to elevate your app’s UI to unprecedented heights. Whether meticulously honing the appearance of a single component or weaving a consistent design language across your app’s entirety, SwiftUI modifiers equip you with the flexibility and expressiveness requisite to actualize your aspirations. Dive into the world of SwiftUI Modifiers, unlock your app’s full potential, and weave a tapestry of innovation within the realm of user interfaces.